一、Vue
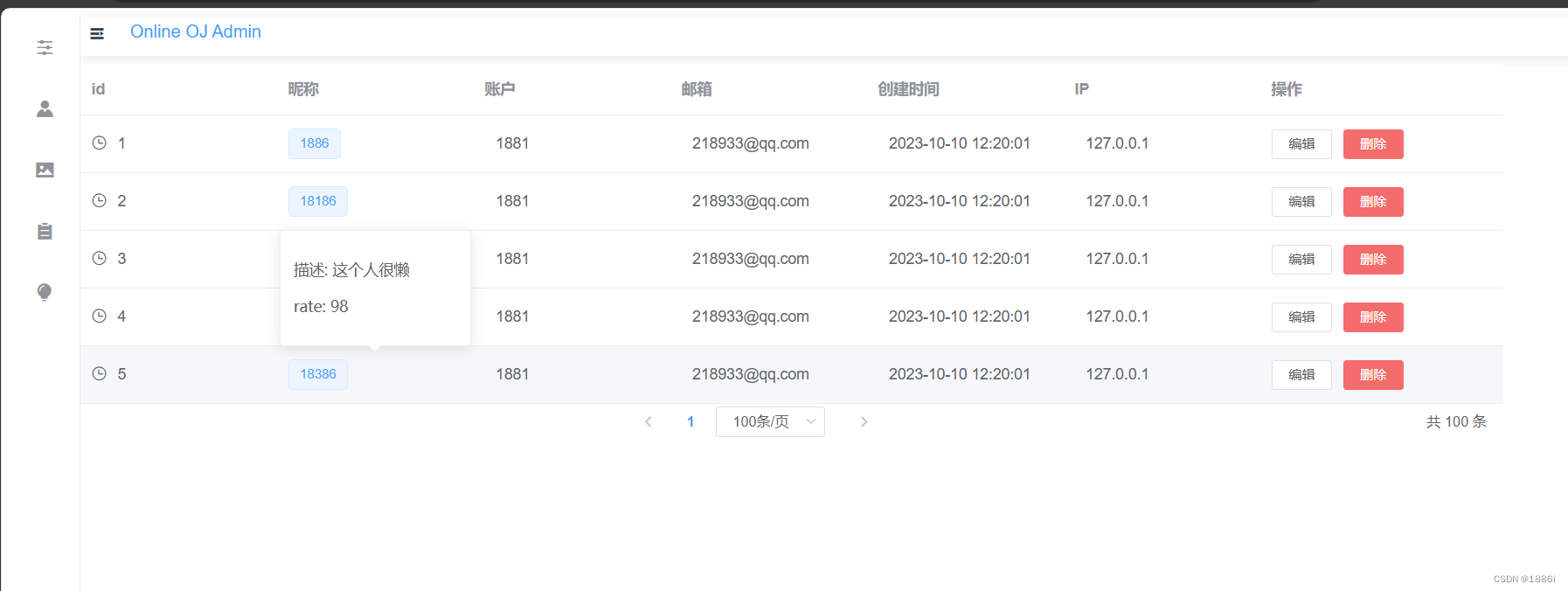
<template>
<div>
<el-table
:data="user"
style="width: 120%">
<el-table-column
label="id"
width="180">
<template slot-scope="scope">
<i class="el-icon-time"></i>
<span style="margin-left: 10px">{{ scope.row.id }}</span>
</template>
</el-table-column>
<el-table-column
label="昵称"
width="180">
<template slot-scope="scope">
<el-popover trigger="hover" placement="top">
<p>描述: {{ scope.row.description }}</p>
<p>rate: {{ scope.row.rating }}</p>
<div slot="reference" class="name-wrapper">
<el-tag size="medium">{{ scope.row.nickname }}</el-tag>
</div>
</el-popover>
</template>
</el-table-column>
<el-table-column
label="账户"
width="180">
<template slot-scope="scope">
<span style="margin-left: 10px">{{ scope.row.account }}</span>
</template>
</el-table-column>
<el-table-column
label="邮箱"
width="180">
<template slot-scope="scope">
<span style="margin-left: 10px">{{ scope.row.email }}</span>
</template>
</el-table-column>
<el-table-column
label="创建时间"
width="180">
<template slot-scope="scope">
<span style="margin-left: 10px">{{ scope.row.createTime }}</span>
</template>
</el-table-column>
<el-table-column
label="IP"
width="180">
<template slot-scope="scope">
<span style="margin-left: 10px">{{ scope.row.ip }}</span>
</template>
</el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button
size="mini"
@click="handleEdit(scope.$index, scope.row)">编辑</el-button>
<el-button
size="mini"
type="danger"
@click="handleDelete(scope.$index, scope.row)">删除</el-button>
</template>
</el-table-column>
</el-table>
<el-pagination
:total="total"
:page-size="pageSize"
:current-page="currentPage"
layout="prev, pager, sizes, next, ->, total"
@current-change="currentChange"
@size-change="sizeChange">
</el-pagination>
</div>
</template>
<script>
export default {
data() {
return {
user: [
{id: 1, nickname: '1886',description: '这个人很懒', ip: '127.0.0.1', account: '1881', rating: 98,email: '218933@qq.com', createTime: "2023-10-10 12:20:01"},
{id: 2, nickname: '18186',description: '这个人很懒', ip: '127.0.0.1', account: '1881', rating: 98,email: '218933@qq.com', createTime: "2023-10-10 12:20:01"},
{id: 3, nickname: '18816', description: '这个人很懒',ip: '127.0.0.1', account: '1881', rating: 98,email: '218933@qq.com', createTime: "2023-10-10 12:20:01"},
{id: 4, nickname: '18826',description: '这个人很懒', ip: '127.0.0.1', account: '1881', rating: 98,email: '218933@qq.com', createTime: "2023-10-10 12:20:01"},
{id: 5, nickname: '18386', description: '这个人很懒',ip: '127.0.0.1', account: '1881', rating: 98,email: '218933@qq.com', createTime: "2023-10-10 12:20:01"},
],
// 当前页码
currentPage: 1,
// 一页数据
pageSize: 10,
// 数据总数
total: 100
}
},
mounted() {
this.getData() // 页码加载获取数据
},
methods: {
getData() {
alert("发起http请求获取数据")
},
handleEdit(index, row) {
this.$message.warning(index + ":edit:" + row)
console.log(index, row);
},
handleDelete(index, row) {
this.$message.warning(index + ":delete:" + row)
console.log(index, row);
},
//页面改变 发起请求获取分页数据
currentChange: function (page) {
this.currentPage = page;
this.$message({
message: '查询页:' + page,
type: 'success'
});
this.getData()
},
// 页面展示数据条数改变 发起请求获取数据
sizeChange: function (size) {
this.pageSize = size;
this.$message({
message: '查询条数:' + this.pageSize,
type: 'success'
});
this.getData()
},
},
components: {
}
}
</script>
二、SpringBoot
PageHelper.startPage(queryVo.getPage(), queryVo.getSize());
PageInfo<ProblemsDTO> problemsDTOPageInfo = new PageInfo<>(res);
problemsDTOPageInfo.setTotal(res.size());
return problemsDTOPageInfo;